This post is about how to auto-wire a set of beans to a list in Spring
. Essentially, we need to add @bean
‘s to an list.
In vanilla Java, we’ll do something like:
1 2 3 4 5 6 7 8 | Person pMike = new Person(); Person pJohn = new Person(); Person pPhilip = new Person(); List<Person> listOfPersons = new ArrayList<>(); listOfPersons.add(pMike); listOfPersons.add(pJohn); listOfPersons.add(pPhilip); |
Requirements
Stuff used in this post.
- IntelliJ IDEA Ultimate 2016.3
- Built-in Spring Initialzr will be used. Alternatively, start.spring.io can be used.
- Spring Boot 1.5.4.RELEASE
- Java 8
- Windows 10 Enterprise
New Maven Project
[wp_ad_camp_1]
Use Spring Initilzr to generate a template Maven project that contains, importantly, the minimum dependencies required to get the application running. This can be done via the built-in Spring Initilzr in IntelliJ or via start.spring.io
In IntelliJ
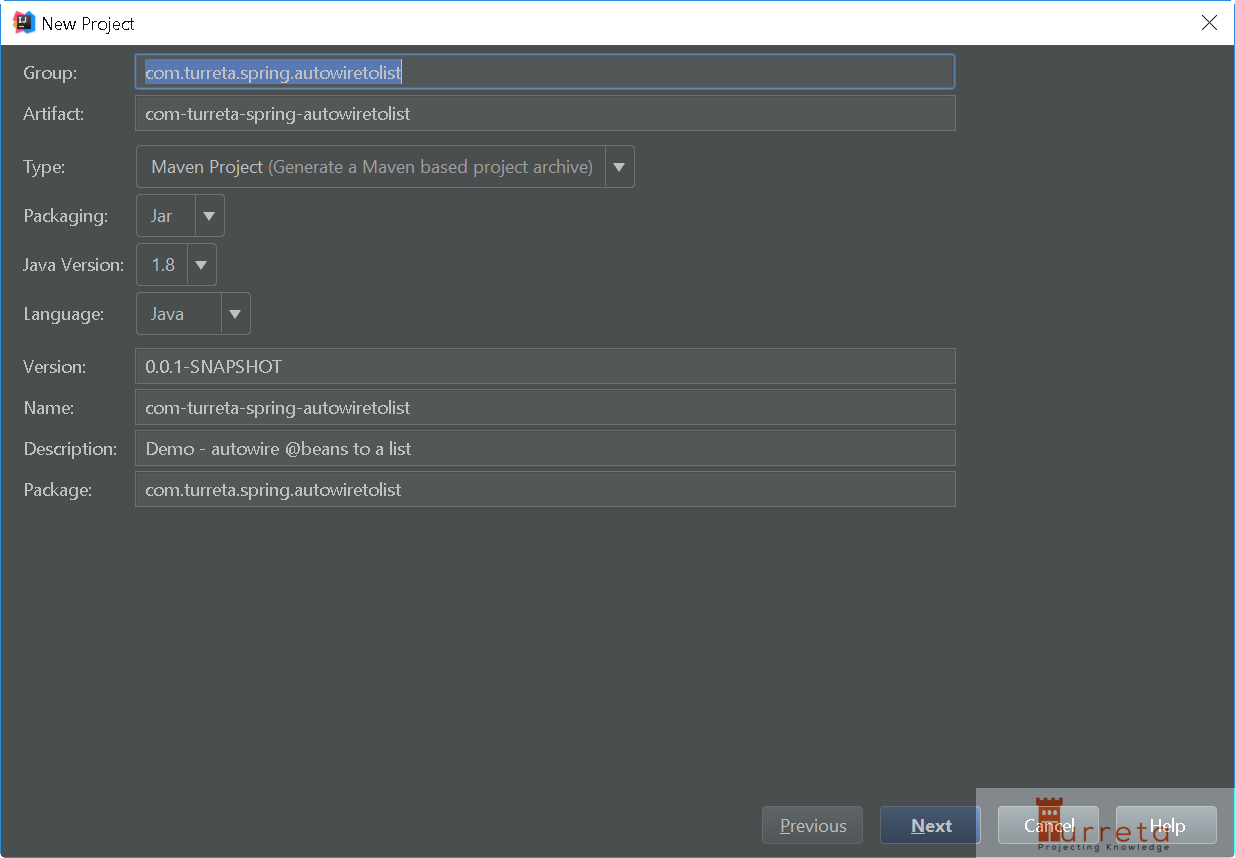
Basic project information for Maven
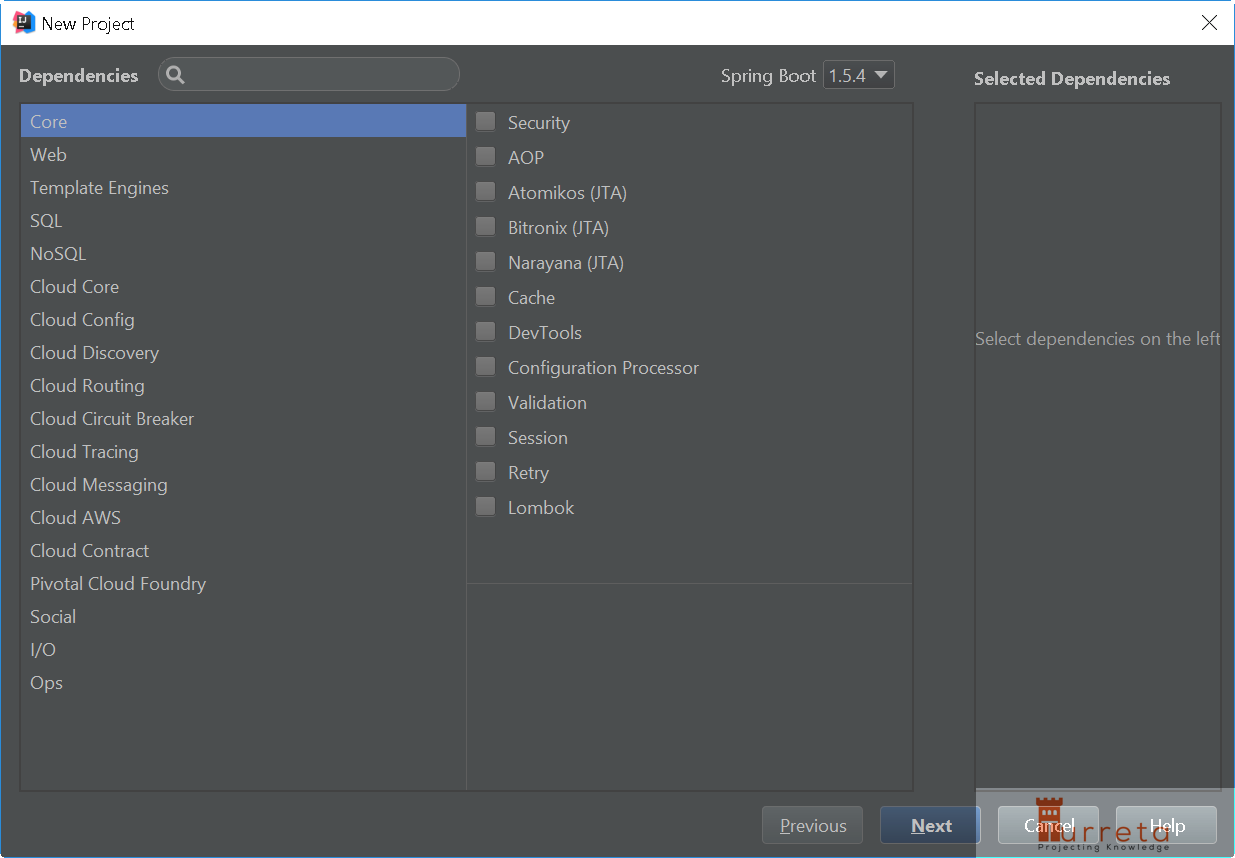
Select nothing. Click Next.
Spring Initlzr – web
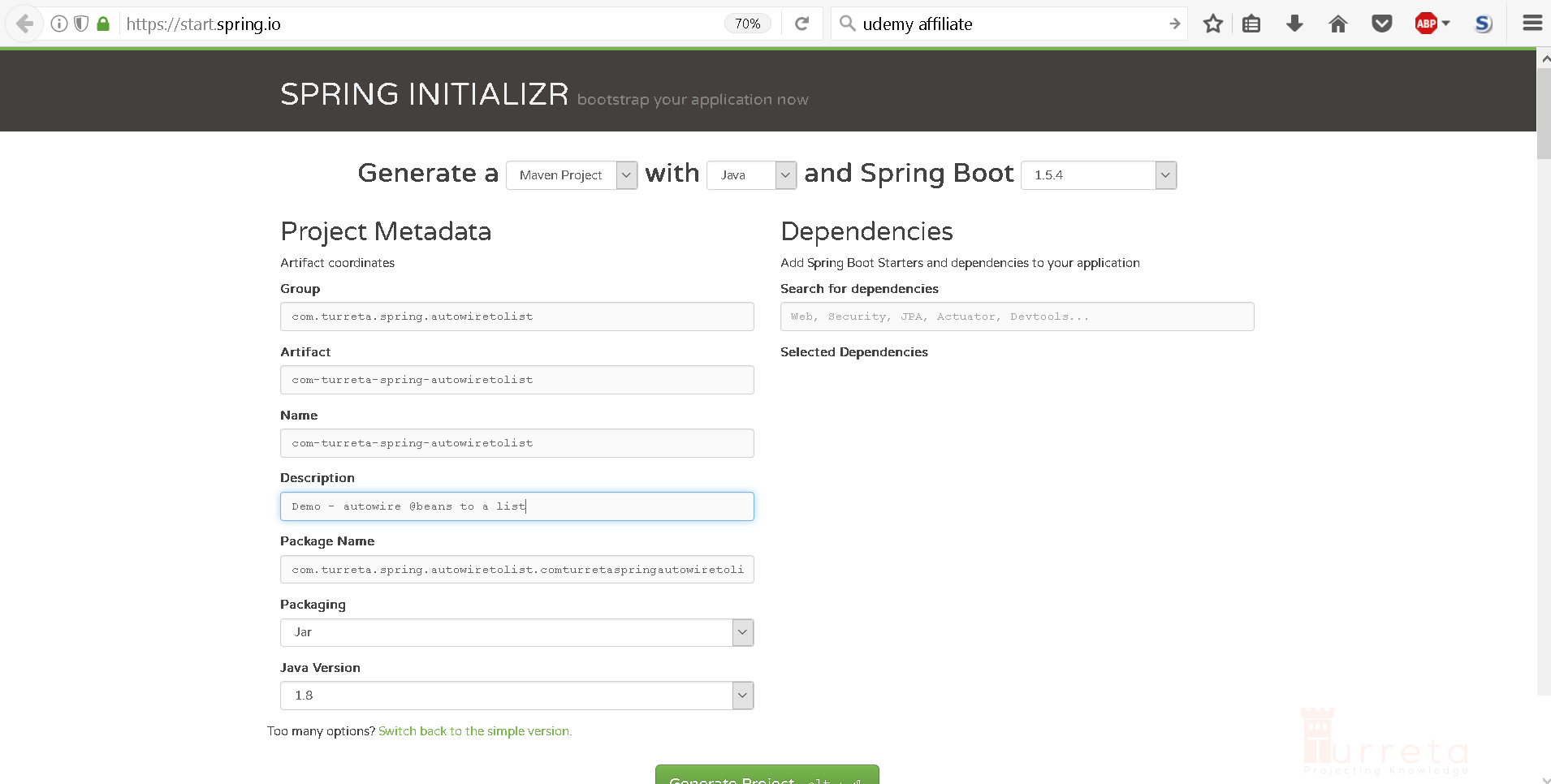
Generate application via https://start.spring.io/
Generated pom.xml
[wp_ad_camp_2]
This is the pom.xml generated from the Spring Initilzr. There’s no need to modify this and we can immediately concentrate on writing our codes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 | <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.turreta.spring.autowiretolist</groupId> <artifactId>com-turreta-spring-autowiretolist</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>com-turreta-spring-autowiretolist</name> <description>Demo - autowire @beans to a list</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.4.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project> |
Java Codes
In SpringBoot, all components get @ComponentScan
‘d automatically when they are located in the same package (including subpackages) as the class that is annotated with @SpringBootApplication
.
Therefore, our codes are in the same package as ComTurretaSpringAutowiretolistApplication
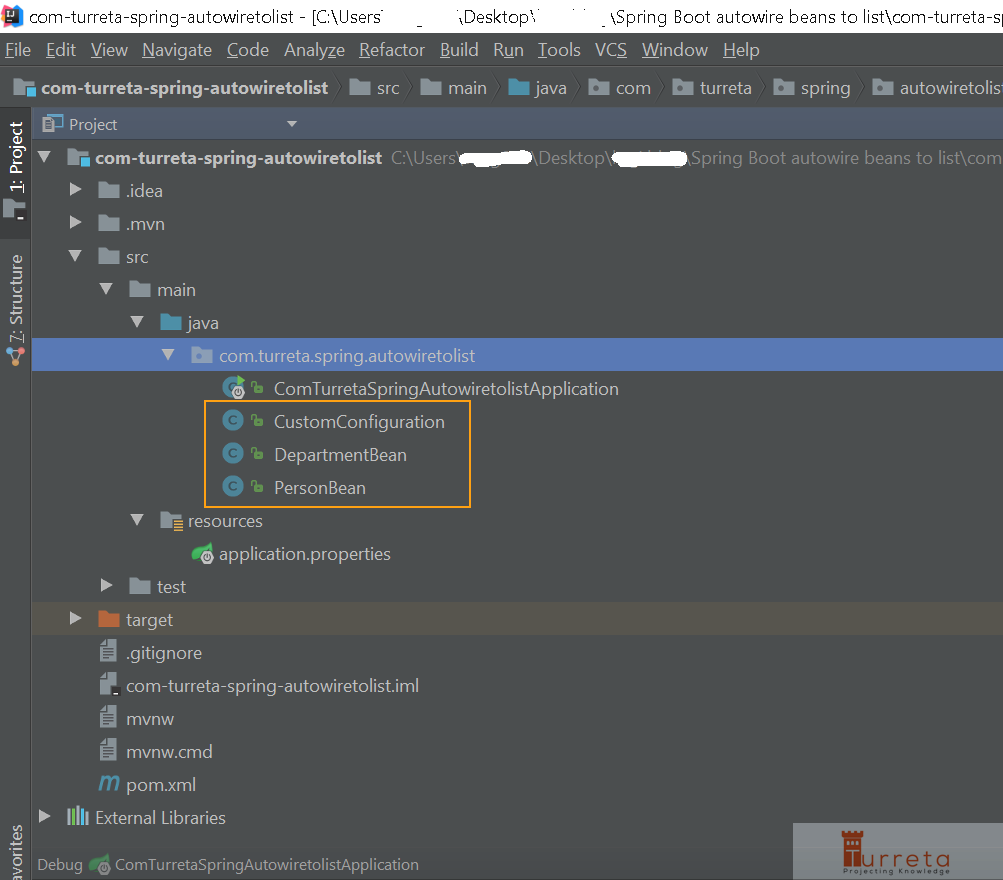
All components are in the same package as the main class
ComTurretaSpringAutowiretolistApplication
This is our main class and Spring Boot application.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | package com.turreta.spring.autowiretolist; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.context.ApplicationContext; @SpringBootApplication public class ComTurretaSpringAutowiretolistApplication { public static void main(String[] args) { ApplicationContext context = SpringApplication.run( ComTurretaSpringAutowiretolistApplication.class, args); DepartmentBean dept = context.getBean("departmentBean", DepartmentBean.class); for(PersonBean person: dept.getListOfPersons()) { System.out.println(person.toString()); } } } |
DepartmentBean
This class represents a Department in a company. A department may contain on or more employees (persons). It is annotated with @Component to allow Spring to instantiate it. Note we have a list field annotated with @Autowired. We need the next 2 classes.
[wp_ad_camp_4]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | package com.turreta.spring.autowiretolist; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; import java.util.List; /** * Created by Turreta.com on 8/7/2017. */ @Component public class DepartmentBean { @Autowired private List<PersonBean> listOfPersons; private String departmentName; public List<PersonBean> getListOfPersons() { return listOfPersons; } public void setListOfPersons(List<PersonBean> listOfPersons) { this.listOfPersons = listOfPersons; } public String getDepartmentName() { return departmentName; } public void setDepartmentName(String departmentName) { this.departmentName = departmentName; } } |
PersonBean
This class represents are person (or employee) assign to a department in a company. Nothing special about it. It’s just a POJO. It is not annotated with anything though. We’ll create instances of this class using the next Java class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 | package com.turreta.spring.autowiretolist; /** * Created by Turreta.com on 8/7/2017. */ public class PersonBean { private String personId; private String lastName; private String firstName; public PersonBean(String personId, String lastName, String firstName) { this.personId = personId; this.lastName = lastName; this.firstName = firstName; } public String getPersonId() { return personId; } public void setPersonId(String personId) { this.personId = personId; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } @Override public String toString() { return "PersonBean{" + "personId='" + personId + '\'' + ", lastName='" + lastName + '\'' + ", firstName='" + firstName + '\'' + '}'; } } |
CustomConfiguration
This class is annotated with @Configuration that will enable us to create several instance of PersonBean using @Bean. The @Order indicates the order which the beans are created and added to the list in the DepartmentBean object.
In the DepartmentBean object, person 1 appears before person 2 and so on.
[wp_ad_camp_5]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | package com.turreta.spring.autowiretolist; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.core.annotation.Order; /** * Created by Turreta.com on 8/7/2017. */ @Configuration public class CustomConfiguration { @Bean @Order(1) public PersonBean a() { return new PersonBean("1", "Duterte", "Rodrigo"); } @Bean @Order(2) public PersonBean b() { return new PersonBean("2", "Dela Rosa", "Ronald"); } @Bean @Order(3) public PersonBean c() { return new PersonBean("3", "Poe", "Grace"); } @Bean @Order(4) public PersonBean d() { return new PersonBean("4", "Ko", "Bong"); } } |
Testing
To test our codes within IntelliJ, simply run ComTurretaSpringAutowiretolistApplication.
Download the Codes