This post demonstrates how to clone objects in Java with Generics using Apache Commons Lang ObjectUtils.
ObjectUtils
[wp_ad_camp_1]
ObjectUtils is utility class from Apache Foundation that can be used to clone objects in Java. It is part of a distributable library called Apache Commons Lang.
One of its methods that is of interest to us is the static method clone(T obj) .
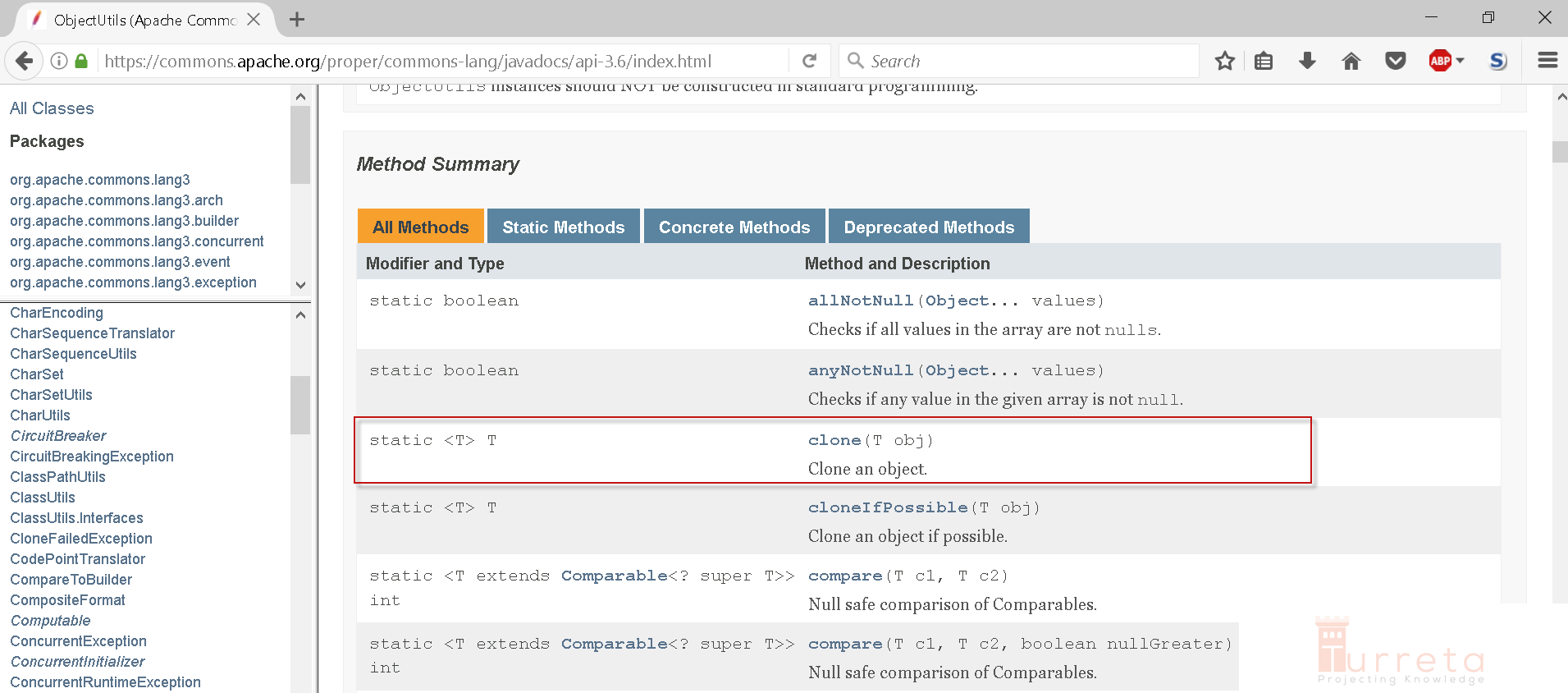
Apache Commons Lang Javadoc
Cloneable Interface
To clone something, it has to be cloneable. Technically speaking, a class has to implement the java.lang.Cloneable and create a non-static no-arg method that calls super.clone() and returns a clone (of type java.lang.Object ) of that class’ object.
For instance:
[wp_ad_camp_2]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | package com.turreta.generics.clone; public class StudentBean implements Cloneable{ private String name; public String getName() { return name; } public void setName(String name) { this.name = name; } // It has to be exactly this method signature public Object clone() { try { // call clone in Object. return super.clone(); } catch(CloneNotSupportedException e) { System.out.println("Unable to clone object"); // Depends on your own use-case. I don't want the object modified somewhere! return null; } } } |
Working with Generics
Okay! Now we have a cloneable class. Let’s create a generic course for students.
[wp_ad_camp_3]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | package com.turreta.generics.clone; import org.apache.commons.lang3.ObjectUtils; public class GenericCourse<T> { private T attendee; public T getAttendee() { return attendee; } public void setAttendee(T attendee) { this.attendee = attendee; } public T cloneAttendee () { return ObjectUtils.clone(attendee); } } |
One line 18, we used ObjectUtils.clone(T object) .
Sample usage:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | package com.turreta.generics.clone; public class CloneDemo { public static void main(String[] args) { GenericCourse<StudentBean> compscie101 = new GenericCourse<>(); StudentBean iStudent = new StudentBean(); iStudent.setName("Karl"); compscie101.setAttendee(iStudent); StudentBean clonedMe = compscie101.cloneAttendee(); System.out.println(iStudent.getName() + " @ " + iStudent); System.out.println(clonedMe.getName() + " @ " + clonedMe); } } |
This outputs:
1 2 | Karl @ com.turreta.generics.clone.StudentBean@7d4991ad Karl @ com.turreta.generics.clone.StudentBean@28d93b30 |
[wp_ad_camp_4]
Note from the output that iStudent and clonedMe objects are different.